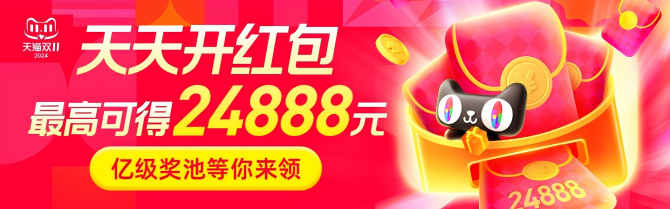
Python是一种强大的编程语言,可以方便地获取网页源代码。下面将从多个方面阐述Python如何获取网页源代码。
1. 使用urllib库获取网页源代码
Python中常用的获取网页源代码的库是urllib库,它包含了一些模块,例如urllib.request、urllib.error、urllib.parse、urllib.robotparser等。
以下是通过urllib库获取网页源代码的示例代码:
import urllib.request url = 'https://www.google.com' response = urllib.request.urlopen(url) html = response.read().decode('utf-8') print(html)
以上代码中,首先通过urllib.request.urlopen()方法打开目标网页,然后使用read()方法读取网页内容,最后使用decode()方法将内容解码成UTF-8格式。
2. 使用requests库获取网页源代码
requests库是Python中的第三方库,也是获取网页源代码的常用库之一。相比于urllib库,requests库更加方便、灵活,支持多种请求方法和请求参数。
以下是通过requests库获取网页源代码的示例代码:
import requests url = 'https://www.google.com' response = requests.get(url) html = response.text print(html)
以上代码中,首先通过requests.get()方法发送GET请求,然后使用text属性获取网页内容。
3. 使用BeautifulSoup库解析网页源代码
当获取到网页源代码后,常常需要对其进行解析,找到目标数据。这时候,就需要用到解析库,其中最常用的是BeautifulSoup库。
以下是通过BeautifulSoup库解析网页源代码的示例代码:
import requests from bs4 import BeautifulSoup url = 'https://www.baidu.com' headers = {'user-agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'} response = requests.get(url, headers=headers) soup = BeautifulSoup(response.text, 'html.parser') title = soup.title.string print(title)
以上代码中,首先通过requests.get()方法发送GET请求,然后使用BeautifulSoup类将返回的网页源代码解析成BeautifulSoup对象,最后使用string属性获取网页标题。
4. 使用Selenium库模拟浏览器获取网页源代码
有时候,直接获取网页源代码会因为目标网站的反爬虫机制而失败,这时候就需要使用Selenium库模拟浏览器,以获取更稳定的数据。
以下是通过Selenium库模拟浏览器获取网页源代码的示例代码:
from selenium import webdriver url = 'https://www.baidu.com' options = webdriver.ChromeOptions() options.add_argument('--headless') options.add_argument('--disable-gpu') options.add_argument('--no-sandbox') driver = webdriver.Chrome(options=options) driver.get(url) html = driver.page_source driver.quit() print(html)
以上代码中,首先通过webdriver.ChromeOptions()方法设置Chrome浏览器参数,然后使用webdriver.Chrome()方法创建Chrome浏览器对象,并通过get()方法模拟访问目标网站,最后使用page_source属性获取网页源代码。
5. 使用代理IP获取网页源代码
当爬取较大量的数据时,单个IP会被目标网站封禁。这时候就需要使用代理IP来解决这个问题。
以下是通过代理IP获取网页源代码的示例代码:
import requests url = 'https://www.google.com' proxy = {'http': 'http://127.0.0.1:8888', 'https': 'https://127.0.0.1:8888'} response = requests.get(url, proxies=proxy, verify=False) html = response.text print(html)
以上代码中,首先通过requests.get()方法发送GET请求,并设置proxy参数为代理IP地址,最后使用verify参数禁用SSL证书验证。
6. 处理网页编码问题
有时候,获取到的网页源代码可能无法正确显示中文字符,这时候就需要对编码进行处理。
以下是通过设置response.encoding属性处理网页编码问题的示例代码:
import requests url = 'https://www.baidu.com' response = requests.get(url) response.encoding = 'utf-8' html = response.text print(html)
以上代码中,首先通过requests.get()方法发送GET请求,然后使用encoding属性设置编码格式,最后使用text属性获取网页内容。
总结
以上就是Python获取网页源代码的介绍,包括使用urllib库、requests库、BeautifulSoup库、Selenium库等方法,以及处理代理IP和编码问题的具体方法。使用这些方法可以更加方便地获取和处理网页源代码,为数据爬取和分析提供了方便。