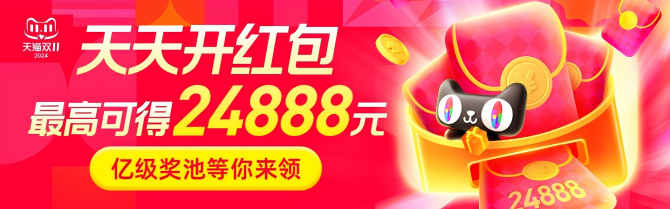
一、状态栏介绍
状态栏是手机屏幕的上部分,一般放置一些系统信息,例如 Wi-Fi 网络连接状态、电池电量信息、时间、手机信号、闹钟等等。
在 iOS 系统中,状态栏总是出现在应用程序的顶部,可用于显示当前设备的网络状态、电量、时间等。状态栏中的图标和文字可能会因设备类型、网络运营商或用户所处的地理区域而有所不同。
在 iOS 13 版本之后,状态栏样式有了一些变化,如果你想自定义状态栏,可以使用UINavigationBar。
二、状态栏样式
状态栏样式是指状态栏文字和图标的颜色和风格,主要由下面两个属性控制:
var statusBarStyle: UIStatusBarStyle { get set } var statusBarAnimation: UIStatusBarAnimation { get set }
第一个属性是 statusBarStyle,它可以设置为以下三种值:
- deafult:默认值,状态栏颜色黑字(白底)或白字(黑底)
- lightContent:状态栏文字为白色
- darkContent:状态栏文字为黑色
第二个属性是 statusBarAnimation,它可以设置为以下三种值:
- none:不进行动画切换
- fade:渐变切换
- slide:滑动切换
你可以通过设置 UIViewController 的 preferredStatusBarStyle 属性和 preferredStatusBarUpdateAnimation 来控制状态栏的颜色和动画切换效果。
override var preferredStatusBarStyle: UIStatusBarStyle { return .lightContent } override var preferredStatusBarUpdateAnimation: UIStatusBarAnimation { return .slide }
三、状态栏隐藏
有些应用场景下可能需要隐藏状态栏,比如当你全屏播放视频、游戏等情况。iOS 系统提供了设置状态栏隐藏状态的方法:
UIApplication.shared.isStatusBarHidden = true // 隐藏状态栏 UIApplication.shared.isStatusBarHidden = false // 显示状态栏
如果你想在应用启动时隐藏状态栏,可以在 AppDelegate 的 didFinishLaunchingWithOptions 方法中添加以下代码:
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool { // 隐藏状态栏 UIApplication.shared.isStatusBarHidden = true return true }
四、状态栏点击事件处理
在 iOS 13 之前,可以通过 UIApplicationDelegate 的 application(_:didFinishLaunchingWithOptions:) 方法来给状态栏添加点击事件。例如:
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool { let tapGesture = UITapGestureRecognizer(target: self, action: #selector(statusBarTapped)) UIApplication.shared.statusBar.addGestureRecognizer(tapGesture) return true } @objc func statusBarTapped() { // 处理状态栏点击事件 }
在 iOS 13 之后,可以使用 scene(_:willConnectTo:options:) 方法在状态栏上添加 UITapGestureRecognizer:
func scene(_ scene: UIScene, willConnectTo session: UISceneSession, options connectionOptions: UIScene.ConnectionOptions) { guard let windowScene = (scene as? UIWindowScene) else { return } let tapGesture = UITapGestureRecognizer(target: self, action: #selector(statusBarTapped)) if let statusBarFrame = windowScene.statusBarManager?.statusBarFrame { let statusBarView = UIView(frame: statusBarFrame) statusBarView.addGestureRecognizer(tapGesture) window?.addSubview(statusBarView) } } @objc func statusBarTapped() { // 处理状态栏点击事件 }
五、状态栏背景颜色
iOS 13 之后,如果你想要自定义状态栏样式,可以使用 UINavigationBar 来设置状态栏样式。比如,如果你想要更改状态栏的背景颜色,可以在 UINavigationBar 中设置。
let navBarAppearance = UINavigationBarAppearance() navBarAppearance.configureWithOpaqueBackground() navBarAppearance.backgroundColor = .red navigationController?.navigationBar.scrollEdgeAppearance = navBarAppearance navigationController?.navigationBar.standardAppearance = navBarAppearance
如果你想要更改状态栏的文字颜色,可以在 UINavigationBar 的 titleTextAttributes 属性中设置。
let navBarAppearance = UINavigationBarAppearance() navBarAppearance.configureWithOpaqueBackground() navBarAppearance.titleTextAttributes = [.foregroundColor: UIColor.white] navigationController?.navigationBar.scrollEdgeAppearance = navBarAppearance navigationController?.navigationBar.standardAppearance = navBarAppearance
六、总结
状态栏是一个非常重要的 UI 组件,它可以让用户在应用程序中方便地获取到一些系统和应用程序的信息。在 iOS 开发中,通过修改状态栏的样式、隐藏、添加点击事件以及背景颜色等属性,可以为用户提供更好的体验。