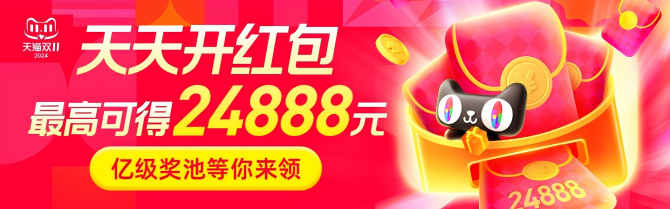
本文将通过介绍Python身份证识别的源码实现方式,分享一些身份证识别相关的知识点。身份证是一种重要的身份证明,而身份证在很多场景下都需要进行识别、验证,实现身份证识别功能可以方便快捷地应用于人脸识别、人员进出场管理等领域。
一、使用Python识别身份证的实现方式
Python语言可以针对身份证的特征进行编程,通过图像处理算法、OCR(Optical Character Recognition,光学字符识别)进行身份证信息的提取和识别。下面是Python身份证识别的实现方式:
import cv2
import numpy as np
import pytesseract
#读取身份证图片
img = cv2.imread("id_card.jpg")
#图像处理,提高身份证信息的识别率
#......
#身份证信息截取
id_number_area = img[400:480, 170:530]
#身份证号码信息OCR识别
id_number = pytesseract.image_to_string(id_number_area, lang='chi_sim')
print("身份证号码:", id_number)
二、图像处理方法提高识别率
由于身份证图像存在光线、角度以及拍摄质量等因素的影响,因此需要对身份证图像进行预处理,以提高身份证信息的识别率。常用的图像处理方式有以下几种:
1、图像灰度化
将彩色图像转化为灰度图像,提高图像处理速度,以便后续进行二值化处理。
#图像灰度化处理函数
def cvtColor(image):
return cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
2、图像二值化
将图像转化为二值图像,即只有黑和白两种颜色,以便后续进行轮廓检测、处理等。
#图像二值化处理函数
def threshold(image):
return cv2.threshold(image, 0, 255, cv2.THRESH_BINARY | cv2.THRESH_OTSU)[1]
3、轮廓检测
通过检测图像的轮廓获取图像的形状信息,以便后续提取身份证信息。
#轮廓检测函数
def findContours(image):
contours, hierarchy = cv2.findContours(image, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
return contours
三、身份证信息提取
通过对图像进行预处理和轮廓检测,可以提取到身份证的各项信息,包括身份证号码、姓名、性别、民族、住址、生日等。在身份证信息提取过程中,我们主要通过OCR技术进行文字识别,通过身份证图片中的各项信息来构建身份证信息。
#通过轮廓检测获取身份证信息的位置
def id_card_ROI(image, contours):
for i in range(len(contours)):
#筛选身份证号码位置信息
if cv2.contourArea(contours[i]) > 1000 and cv2.contourArea(contours[i]) 100 and h > 10:
#通过OCR技术进行身份证号码信息识别
id_number_area = image[y: y + h, x: x + w]
id_number = pytesseract.image_to_string(id_number_area, lang='chi_sim')
return id_number_area
四、完整代码示例
下面是Python身份证识别的完整代码示例:
import cv2
import numpy as np
import pytesseract
#图像灰度化处理函数
def cvtColor(image):
return cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
#图像二值化处理函数
def threshold(image):
return cv2.threshold(image, 0, 255, cv2.THRESH_BINARY | cv2.THRESH_OTSU)[1]
#轮廓检测函数
def findContours(image):
contours, hierarchy = cv2.findContours(image, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
return contours
#通过轮廓检测获取身份证信息的位置
def id_card_ROI(image, contours):
for i in range(len(contours)):
#筛选身份证号码信息
if cv2.contourArea(contours[i]) > 1000 and cv2.contourArea(contours[i]) 100 and h > 10:
#身份证号码区域截取
id_number_area = image[y: y + h, x: x + w]
#身份证号码OCR识别
id_number = pytesseract.image_to_string(id_number_area, lang='chi_sim')
return id_number_area
#读取身份证图片
img = cv2.imread("id_card.jpg")
#图像灰度化
gray_img = cvtColor(img)
#图像二值化
threshold_img = threshold(gray_img)
#轮廓检测
contours = findContours(threshold_img)
#身份证信息截取和OCR识别
id_number_area = id_card_ROI(img, contours)
#打印结果
print("身份证号码:",id_number)
五、总结
Python身份证识别是一项常用的图像处理技术,通过预处理、轮廓检测、OCR技术提取并识别身份证信息。在实现身份证识别过程中,需要注意图片质量、处理方法、算法优化等方面,以提高身份证识别的准确率。同时,针对不同的应用场景和需求,可以对身份证识别的算法和方法进行进一步的优化和改进。