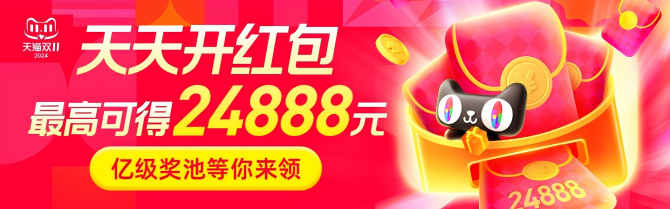
#include <stdio.h> //基本类型 typedef int MyInt; //可以对typedef产生的类型名二次起别名 typedef MyInt MyInt2; // 给指针类型char *起一个新的类型名称String typedef char * String; //给结构体定义别名 //方法一:先定义类型,再声明别名 struct Student1 { int age; }; typedef struct Student1 MyStu1; //方法二:定义类型的同时声明别名 typedef struct Student2 { int age; } MyStu2; //方法三:定义类型的同时声明别名,此时省略了结构体名称 typedef struct { int age; } MyStu3; //使用枚举的方法和结构体类似 //定义结构体指针 //方法一:先定义类型,在定义指针别名 struct Student4 { int age; }; typedef struct Student4 *MyStu4; //方法二:定义类型的同时定义指针别名 typedef struct Student5 { int age; } *MyStu5; //使用typedef定义函数指针类型的别名,此时MyPoint就是函数指针类型 typedef void *MyPoint)int, int); void testint a, int b); int main) { /************************************************************* * typedef:给已经存在的类型起一个新的名称,该类型可以是系统类型,也可以是自定义类型 * 2.使用场合: * 1、基本数据类型 * 2、指针 * 3、结构体 * 4、枚举 * 5、指向函数的指针 * 注意:使用typedef产生的类型和元类型的作用一样 * typedef的某些功能使用宏定义也可以实现但是,宏定义仅仅只是替换,使用不当会发生错误 *************************************************************/ MyInt a = 10; printf"a = %d ", a); MyInt2 b = 10; printf"b = %d ", b); String str = "abc"; printf"str = %s ", str); MyStu1 stu1 = {20}; MyStu2 stu2 = {21}; MyStu3 stu3 = {22}; printf"stu1.age = %d ", stu1.age); printf"stu2.age = %d ", stu2.age); printf"stu3.age = %d ", stu3.age); struct Student4 Stu4 = {23}; MyStu4 pstu4 = &Stu4; printf"stu4.age = %d ", pstu4->age); struct Student5 Stu5 = {24}; MyStu5 pstu5 = &Stu5; printf"stu5.age = %d ", pstu5->age); MyPoint p ; p = test; p5, 10); return 0; } void testint a, int b) { printf"a + b = %d ", a + b); return; }
a = 10 b = 10 str = abc stu1.age = 20 stu2.age = 21 stu3.age = 22 stu4.age = 23 stu5.age = 24 a + b = 15